package paineis;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
/**
*
* @author HENRIQUE
*/
public class BotaoPainel extends Application{
StackPane painel = new StackPane();
@Override
public void start(Stage stage) throws Exception {
painel.getChildren().add(new Button("Clique aqui"));
Scene scene = new Scene(painel, 200, 50);
stage.setTitle("Botão dentro de um painel");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
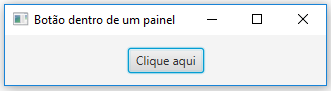
EXEMPLO 2: Circulo
package paineis;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
/**
*
* @author HENRIQUE
*/
public class Circulo extends Application{
@Override
public void start(Stage stage){
Circle circle = new Circle();
circle.setCenterX(100);//cpordenada
circle.setCenterY(100);//coordenada
circle.setRadius(50);
circle.setStroke(Color.BLACK);
circle.setFill(Color.WHITE);
Pane pane = new Pane();
pane.getChildren().add(circle);
Scene scene = new Scene(pane, 200, 200);
stage.setTitle("Exibindo um circulo");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
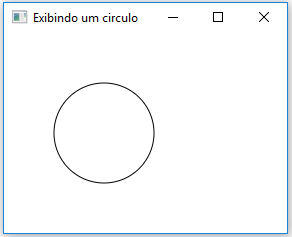
EXEMPLO 3: Circulo centralizado
package paineis;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
/**
*
* @author HENRIQUE
*/
public class CirculoCentralizado extends Application{
@Override
public void start(Stage stage){
Pane pane = new Pane();
Circle circle = new Circle();
circle.centerXProperty().bind(pane.widthProperty().divide(2));
circle.centerYProperty().bind(pane.heightProperty().divide(2));
circle.setRadius(50);
circle.setStroke(Color.BLACK);
circle.setFill(Color.WHITE);
//circle.setFill(Color.RED);
pane.getChildren().add(circle);
Scene scene = new Scene(pane, 200, 200);
stage.setTitle("Circulo Sempre Centralizado");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}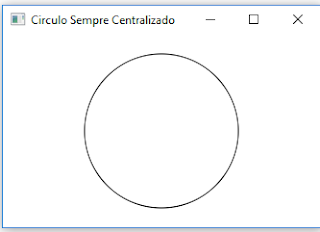
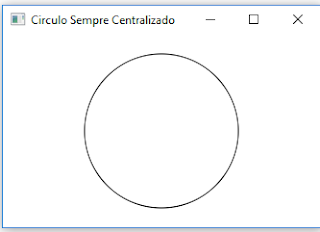
EXEMPLO 4: Interface com JavaFX
package paineis;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
/**
*
* @author HENRIQUE
*/
public class MinhaPrimeiraInterface extends Application {
@Override
public void start (Stage primaryStage){
Button btn = new Button("Sou um Botão");
Scene scene = new Scene(btn, 200, 250);
primaryStage.setTitle("Minha Primeira Interface JavaFX");
primaryStage.setScene(scene);
primaryStage.show();
Stage stage = new Stage();
stage.setTitle("Nova janela");
stage.setScene(new Scene(new Button("Mais um botão"),100, 100));
stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
EXEMPLO 5: Interface dentro de uma interface
package paineis;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
/**
*
* @author HENRIQUE
*/
public class PainelDentroDePainel extends Application{
@Override
public void start(Stage stage) {
BorderPane painelPrincipal = new BorderPane();
FlowPane painelSul = new FlowPane();
painelSul.getChildren().add(new Button("Botão 1"));
painelSul.getChildren().add(new Button("Botão 2"));
painelPrincipal.setBottom(painelSul);
Scene scene = new Scene(painelPrincipal, 400, 400);
stage.setTitle("Painel dentro de um Painel");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
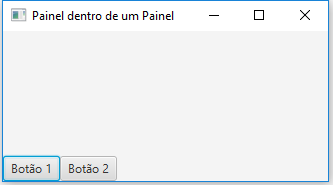
Nenhum comentário:
Postar um comentário